One of the things I like when reading text files on unity that contains data I need for whatever the thing i’m making is the TextAsset.
Start with a simple script like this:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class TextAssetExample : MonoBehaviour
{
public TextAsset myFile;
void Start()
{
}
void Update()
{
}
}
And you can use the myFile field to place whatever the text asset you want.
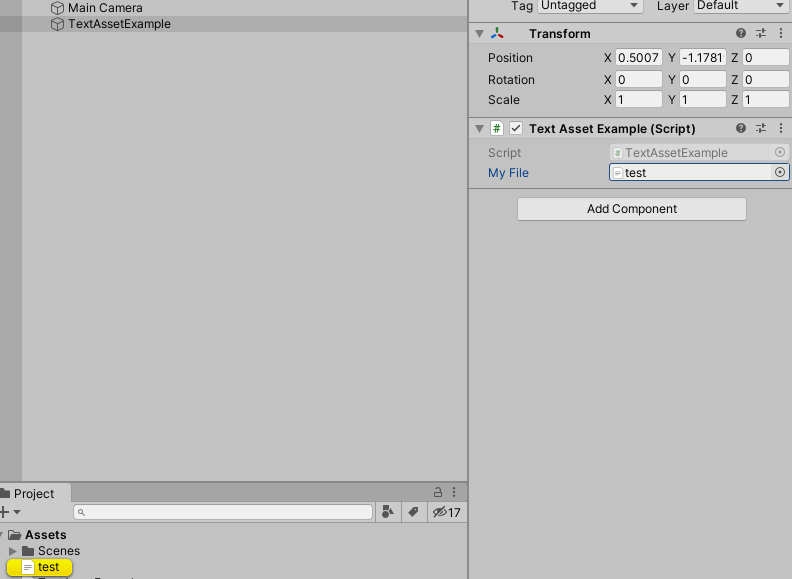
I will fill in the text file with anything just for the example.
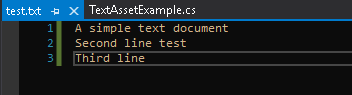
You can grab very useful properties from the file through the TextAsset object like it’s name, content (literally a string containing all the file’s content), the raw bytes and the size of it.
void Start()
{
string name = myFile.name;
string content = myFile.text;
byte[] rawBytes = myFile.bytes;
int size = myFile.bytes.Length;
Debug.Log("The file text " + name + " has a size of " + size + " bytes");
Debug.Log("The file content: \n" + content);
}
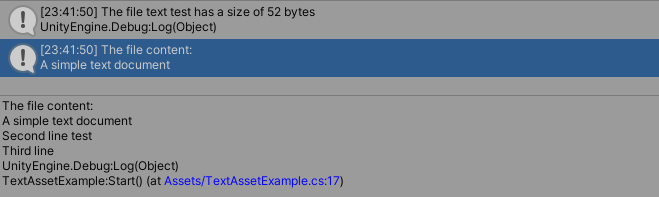
The cool thing about it is that you can move the asset file to any folder you want and change the location of it because it is an object field on the script, you don’t need to always know the path of it to load using File.ReadAllText or something else.
And that was it just a little cool tip. Here is the code: